Building a Jamstack Blog with Next.js, WordPress, and Cloudinary
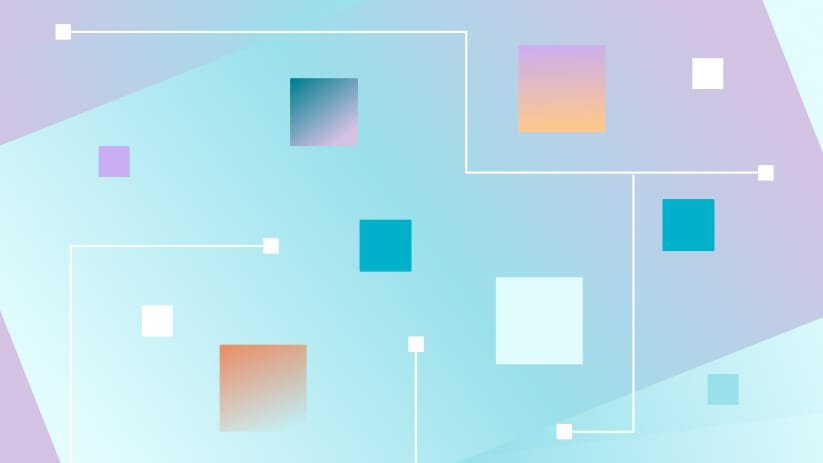
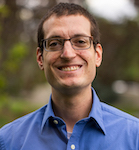
This example showcases the Next.js Static Generation feature using a GraphQL API built on StepZen to query the data sources -- WordPress is the CMS backend to hold our blog posts, and Cloudinary the source for images. We use Next.js to statically generate the posts.
To get started, sign in to your StepZen Account. If you don't have an account you can sign up for free . You can view a demo on Vercel and the repository at StepZen Samples.
1. Prepare your WordPress site
Inside your WordPress admin, go to Posts and start adding new posts. Only published posts and public fields will be rendered.
- Create at least 2 posts with dummy data for content
- Pick an author from your WordPress users
- Add a Featured Image. You can download one from Unsplash
- Fill the Excerpt field
- When you’re done, make sure to Publish the posts
2. Create a Schema for Combining WordPress and Cloudinary Data with StepZen Import
StepZen makes it easy to build a single GraphQL API that combines data from any backend data source in a unified API. StepZen comes with API Templates that are pre-made GraphQL schemas configured with types, queries, and backends, designed to be combined, customized, and extended and help you quickly create your GraphQL API.
The stepzen import
command imports one or more template schemas to start your project. API Templates are pre-made GraphQL schemas configured with types, queries, and backends, designed to be combined, customized, and extended and give you a jumpstart creating your unified GraphQL API. In this case, we import the WordPress
and Cloudinary
templates, which can be viewed on our StepZen Schemas repository.
Install stepzen
CLI and create project directory
npm install -g stepzen
mkdir wp-stepzen && cd wp-stepzen
Import WordPress Template
stepzen import wordpress
For WordPress you will be asked to include your WordPress username
and password
.
What is the wordpress username? [hidden]
What is the wordpress password? [hidden]
Import Cloudinary Template
stepzen import cloudinary
For Cloudinary you will be asked to include your Cloudinary api_key
, api_secret
, and cloud_name
.
What is the api_key for your cloudinary API? [hidden]
What is the api_secret for your cloudinary API? [hidden]
What is the cloud_name for your cloudinary API? [hidden]
config.yaml
This will generate a config.yaml
file containing the keys and other credential information that StepZen needs to access your backend data sources. This file should be added to .gitignore
as it contains secret information.
configurationset:
- configuration:
name: wordpress_config
username: { { username } }
password: { { password } }
- configuration:
name: cloudinary_config
api_key: { { api_key } }
api_secret: { { api_secret } }
cloud_name: { { cloud_name } }
index.graphql
Every StepZen project requires an index.graphql
. This file defines all the files that make up the unified GraphQL schema. If you don't want to include Cloudinary, comment it out.
schema
@sdl(
files: [
"wordpress/posts.graphql"
"wordpress/pages.graphql"
"cloudinary/cloudinary.graphql"
]
) {
query: Query
}
3. Query Your WordPress and Cloudinary Backends
stepzen start
uploads and deploys your GraphQL endpoint while watching the project for changes. In your root folder run:
stepzen start
From the StepZen dashboard, you can view the GraphQL API and test your queries. For example, enter the following query:
{
wordpressPages {
id
title
content
featuredImage
slug
}
wordpressPosts {
id
title
content
featuredImage
slug
}
}
4. Setting up Cloudinary Images
To include images from Cloudinary you will first need to create a Cloudinary account. Once you have created an account you will have access to your Cloudinary Dashboard.
In the top right where it says {{Cloud Name}}, you will see your own cloud name for your config.
Add all the images you want to feature on your pages with the slug of the matching post and page. The images are named to align with the slug of the WordPress page.
- WordPress URL -
https://yoursite.com/post/hello-world
- Image Name (PublicId) -
hello-world
Uncomment the cloudinaryImage
data found on index.js
and [slug].js
for getStaticProps
and coverImage
. Then delete {post.featuredImage}
found on index.js
and [slug].js
and save the project.
5. Next.js Configurations
To configure our Next.js site we will need to create a file to hold our environment variables. Copy the .env.local.example
file in this directory to .env.local
(you will also want to include this in your .gitignore
file):
cp .env.local.example .env.local
Open .env.local
and set STEPZEN_API_URL
to be the URL to your GraphQL endpoint in WordPress. For example: https://{stepzenid}.stepzen.net/tutorials/helloworld/__graphql
.
STEPZEN_API_URL=<YOUR_API_URL>
STEPZEN_AUTH_REFRESH_TOKEN=<YOUR_REFRESH_TOKEN>
Run Next.js in development mode
To run Next.js in development mode with npm
, enter the following command.
npm i && npm run dev
If you are using yarn
instead of npm
, enter the following command.
yarn && yarn dev
Your blog should be up and running on http://localhost:3000! If it doesn't work, leave us a note on GitHub Issues.
6. Deploy on Vercel
You can deploy this app to the cloud by pushing it to GitHub/GitLab/Bitbucket and importing to Vercel.
For more detailed setup instructions you can checkout the README on the stepzen-wordpress-nextjs repo at the StepZen Github. You can also checkout a deployed example at next-blog-wordpress.now.sh.